7장 보고 정리하기.. -> 과제 구글링으로 정리햇다
메인액티비티
package com.example.aap;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
import android.content.DialogInterface;
import android.os.Bundle;
import android.view.Display;
import android.view.Gravity;
import android.view.View;
import android.view.WindowManager;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
EditText tvName,tvEmail, tvAddress, tvSchool;
EditText dlgEdtName,dlgEdtEmail,dlgEdtSchool,dlgEdtAddress;
TextView toastText;
View dialogView, toastView;
Button btn1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
setTitle("사용자 정보 입력");
tvName=(EditText) findViewById(R.id.tvName);
tvEmail=(EditText) findViewById(R.id.tvEmail);
tvAddress=(EditText) findViewById(R.id.tvAddress);
tvSchool=(EditText) findViewById(R.id.tvSchool);
btn1=(Button)findViewById(R.id.btn1);
btn1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
dialogView=(View)View.inflate(MainActivity.this,R.layout.dialog,null);
AlertDialog.Builder dlg=new AlertDialog.Builder(MainActivity.this);
dlg.setTitle("사용자 정보 입력");
dlg.setIcon(R.mipmap.ic_launcher);
dlg.setView(dialogView);
dlgEdtName=(EditText)dialogView.findViewById(R.id.dianame);
dlgEdtEmail=(EditText)dialogView.findViewById(R.id.diaemail);
dlgEdtSchool=(EditText)dialogView.findViewById(R.id.diaschool);
dlgEdtAddress=(EditText)dialogView.findViewById(R.id.diaaddress);
//에딧텍스트의 문구를 에딧텍스트에 복사하므로 .toString()함수 필요x
dlgEdtName.setText(tvName.getText());
dlgEdtEmail.setText(tvEmail.getText());
dlgEdtAddress.setText(tvAddress.getText());
dlgEdtSchool.setText(tvSchool.getText());
dlg.setPositiveButton("확인", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
tvName.setText(dlgEdtName.getText());
tvEmail.setText(dlgEdtEmail.getText());
tvAddress.setText(dlgEdtAddress.getText());
tvSchool.setText(dlgEdtSchool.getText());
}
});
dlg.setNegativeButton("취소", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Toast toast=new Toast(MainActivity.this);
toastView=(View)View.inflate(MainActivity.this,R.layout.toast1,null);
toastText=(TextView)toastView.findViewById(R.id.textView);
toastText.setText("취소했습니다.");
toast.setView(toastView) ;
Display display=((WindowManager)getSystemService(WINDOW_SERVICE)).getDefaultDisplay();
toast.show();
}
});
dlg.show();
}
});
}
}
액티비티 메인 xml
<?xml version="1.0" encoding="utf-8"?>
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center_horizontal">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/tvName"
android:hint="이름"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/tvEmail"
android:hint="이메일"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/tvAddress"
android:hint="주소"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/tvSchool"
android:hint="학과"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/btn1"
android:text="여기를 클릭"/>
</LinearLayout>
토스트
<?xml version="1.0" encoding="utf-8"?>
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:textSize="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="사용자 이름"/>
<EditText
android:textSize="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/dianame"/>
<TextView
android:textSize="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="이메일"/>
<EditText
android:textSize="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/diaemail"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="학과 입력"
android:textSize="20dp" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/diaschool"
android:textSize="20dp"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="주소 입력"
android:textSize="20dp" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/diaaddress"
android:textSize="20dp"/>
</LinearLayout>
대화상자
<?xml version="1.0" encoding="utf-8"?>
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:textSize="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="사용자 이름"/>
<EditText
android:textSize="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/dianame"/>
<TextView
android:textSize="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="이메일"/>
<EditText
android:textSize="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/diaemail"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="학과 입력"
android:textSize="20dp" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/diaschool"
android:textSize="20dp"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="주소 입력"
android:textSize="20dp" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/diaaddress"
android:textSize="20dp"/>
</LinearLayout>
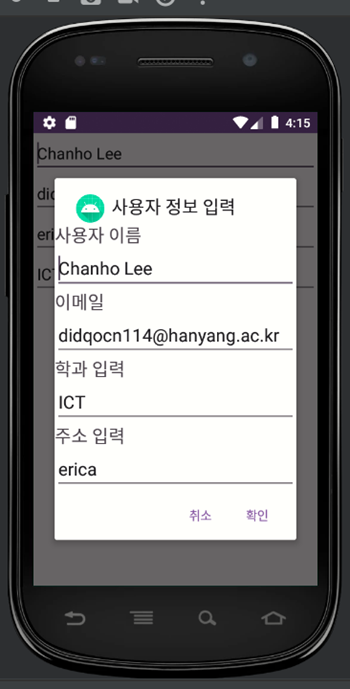
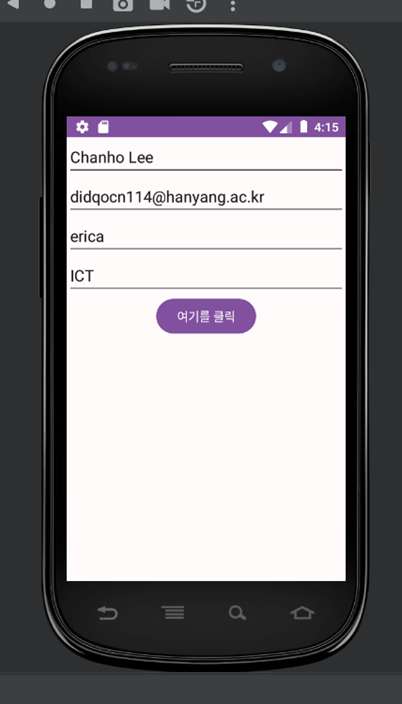
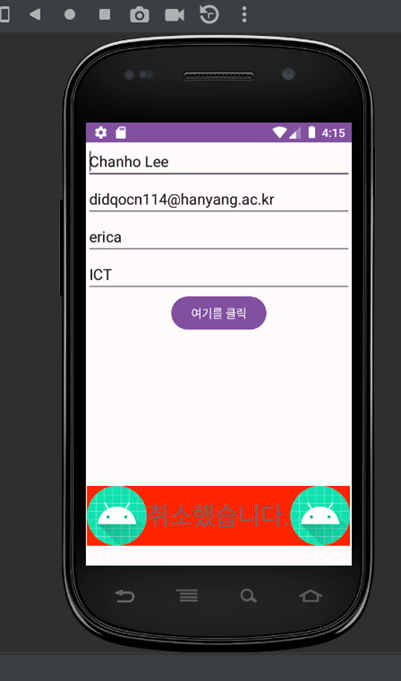